How to apply Git Diff and deal with Patch Failed error
Git diff is a powerful command which allows you to see you recently made changes whether they are staged or not. There are however circumstances when you want to stash your diff on one branch and apply on other. We may also have to stash local changes in case we are going to fetch from remote and merge or rebase with destination branch.
Stashing and applying diff on the same machine is as easy as applying following commands,
git stash
// Do git pull/merge/rebase with destination branch
git stash pop // We can also do git stash apply stash@{0}
However, what if you made changes and want to apply that diff
on someone else's workstation? We can follow the workflow below to apply it on the other machine.
Suppose I have following tracking protocol which houses a protocol method to track the tap,
protocol TrackingProtocol {
func trackTap(event: String)
}
However, I want to add one more method to track click to with click locations. Namely, xLocation
and yLocation
. So I make those changes and run git diff
to verify if they're correct,

These changes are local to my machine. If I want to port them to someone else's workstation, I will need to save them in the diff
file. I chose to save these diffs in file named track-click-location-additions.diff
git diff > ~/Desktop/track-click-location-additions.diff
Once we send this file over to someone else, they can store it on their machine and run git apply
to apply these changes
git apply ~/Desktop/track-click-location-additions.diff
This should be all and if you verify this diff now with git diff
command, you will see that all the changes have been correctly applied on your branch. And that's the end of the happy path story.
What if I run into error: patch failed - patch does not apply
error message while applying patch?
There could be situations where you might run into error saying git failed to apply patch and concerned diff can no longer be applied
<diff file full path>: trailing whitespace.
error: patch failed: <full file path>
error: <full file path>: patch does not apply
According to one of the StackOverflow answers this happens because,
By default, git will warn about whitespace errors, but will still accept them. If they are hard errors then you must have changed some settings
But this is strange. I never changed any settings since last time I diff
'ed and stored it in the local file. However, we can easily overcome this error by adding an extra argument namely --whitespace=warn
git apply --whitespace=warn ~/Desktop/track-click-location-additions.diff
In my case in other example, it warned me because my changes had trailing whitespaces,
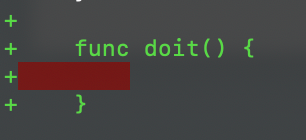
But if I go back and explicitly remove these trailing spaces from original changes, it will stop complaining and outright apply that diff without any warning or complaint,
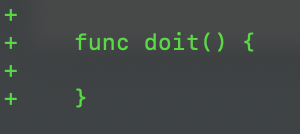
So that should be all for today's post. To summarize, today we saw
- How to apply
diff
patch using Git command - How to overcome
error: patch failed
after applying thediff
- How to deal with trailing whitespace warning message after applying the
diff
References:
StackOverflow - My diff contains trailing whitespace - how to get rid of it?