iOS - Convert Array to String and String to Array in Swift
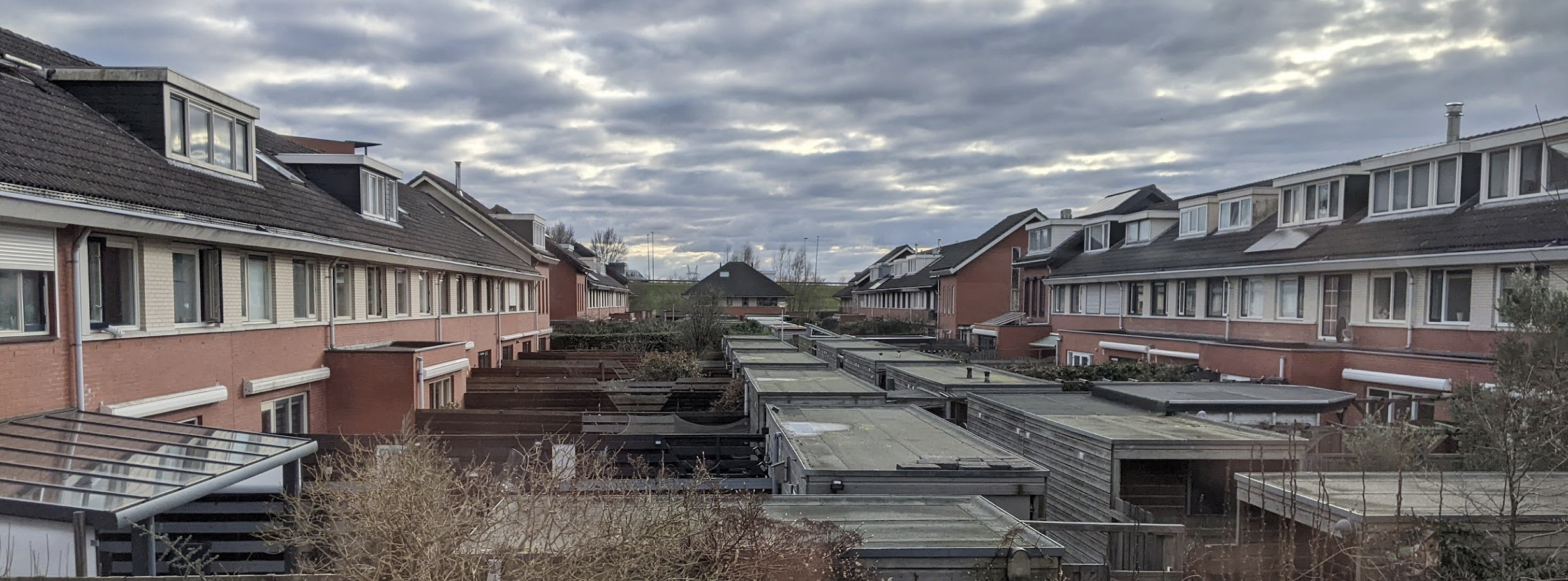
Some of the most frequent operations in iOS involve converting an array to a string and string back to an array. This happens when the user has an array of objects but needs to convert them into the user-facing message, or a long string separated by a fixed delimiter needs to be converted into array-based tokens.
Despite this frequent usage, iOS doesn't have easily memorable names for these APIs. In today's blog post, I am going to talk about standard iOS APIs to convert an array to a string and then string back to the array.
- Converting Array to String in iOS using Swift
To convert a Swift string array to a single String, we are going to use joined
method on Swift arrays. You must use the array of strings to use this method.
This method takes optional separator
parameter. If no separator
parameter is specified, all the array elements are joined without any space between them.
For example,
let names: [String] = ["Bob", "Tom", "Steve"]
let joinedNamesWithSeparator = names.joined(separator: ", ")
print(joinedNamesWithSeparator) // prints Bob, Tom, Steve
let joinedNamesWithoutSeparator = names.joined()
print(joinedNamesWithoutSeparator) // prints BobTomSteve
Since this API only works with string arrays as specified in the documentation below,
// Taken from official Apple documentation
extension Array where Element == String {
public func joined(separator: String = "") -> String
}
you might wonder how to convert an array of custom objects to a string. You can easily do it by mapping an array of custom objects to an array of strings and then calling this API to get a single string back.
Let's understand it with an example below,
let people: [Person] = [Person(firstName: "Top", lastName: "Holland"), Person(firstName: "Doctor", lastName: "Strange"), Person(firstName: "Tony", lastName: "Stark")]
let joinedNamesWithSeparator = people.map { $0.firstName }.joined(separator: ", ")
// prints Top, Doctor, Tony
print(joinedNamesWithSeparator)
let joinedNamesWithoutSeparator = people.map { $0.firstName }.joined()
// prints TopDoctorTony
print(joinedNamesWithoutSeparator)
And that's it. Now you can convert either an array of strings or an array of custom objects to a string.
2. Â Converting String to Array in iOS using Swift
In this next part, we are going to see how to convert a string into an array of strings. To convert single strings into an array of strings, you need to specify the delimiter which will be used to divide a single string into multiple tokens in the form of an array.
For example, consider this -
let namesCollection = "Top, Doctor, Tony"
let namesArray = namesCollection.components(separatedBy: ",")
// Prints ["Top", " Doctor", " Tony"]
print(namesArray)
We had a single string "let namesCollection = "Top, Doctor, Tony"
where each substring was separated by a character ,
. We used components(separatedBy
API to tokenize this string into an array separated by ,
so that each substring separated by ,
became an individual element in the array.
If the separator character does not exist in the string, this API returns an array with a single element which is, the original string back.
let namesCollection = "Top, Doctor, Tony"
let namesArray = namesCollection.components(separatedBy: ":")
// prints ["Top, Doctor, Tony"]
print(namesArray)
Summary
And that's all folks. Next time when you run into converting an array to a string or a string to an array conversion, you can use these APIs as a handy tool. To summarize, here's how you can use them.
let names: [String] = ["Bob", "Tom", "Steve"]
let joinedNamesWithSeparator = names.joined(separator: ", ")
// prints Bob, Tom, Steve
print(joinedNamesWithSeparator)
let namesCollection = "Top, Doctor, Tony"
let namesArray = namesCollection.components(separatedBy: ", ")
// prints ["Top", "Doctor", "Tony"]
print(namesArray)
Support and Feedback
If you have any comments or questions, please feel free to reach out to me on LinkedIn.
If you like my blog content and wish to keep me going, please consider donating on Buy Me a Coffee or Patreon. Help, in any form or amount, is highly appreciated and it's a big motivation to keep me writing more articles like this.
Consulting Services
I also provide a few consulting services on Topmate.io, and you can reach out to me there too. These services include,